Your web browser is out of date. Update your browser for more security, speed and the best experience on this site.
The Browser in the loop
The Event Loop is essential to the interaction between JavaScript and your web pages. Everyone who is developing for web browsers knows what the Event Loop is, but it still leads to numerous issues.
EVERYTHING YOU NEED TO KNOW ABOUT THE EVENT LOOP OF BROWSERS
The Event Loop is essential to the interaction between JavaScript and your web pages. Everyone who is developing for web browsers knows what the Event Loop is, but it still leads to numerous issues. Pages that crash, applications that load annoyingly slowly, elements that move suddenly...this often involves a wrong method of calling on the Event Loop. We will take a look at a few typical scenarios, also thanks to Jake Archibald.
By Joey Comhaire – Java Developer at Axxes.
WHY DOES THE EVENT LOOP OCCUR?
Web pages are structured and managed using a single main thread. The code on the thread is executed in a fixed order to ensure that everything goes smoothly. This leads to a number of challenges. If an element in your thread takes too long to load, the rest of the code will halt as well.
The use of setTimeout in JavaScript is a good example of this. If you use this function to incorporate a break of a few milliseconds, the execution of the rest of your code will also be delayed. Your page will load slower, which leads to a poor user experience. You will preferably have setTimeout wait for a separate thread and subsequently execute the postponed call back on the main thread.
The Event Loop ensures that this process takes place properly by using queues on which you park various types of tasks. The Event Loop processes the elements on these queues in a fixed order. There are three queues:
- The task queue (or call back queue)
- Render steps
- The micro-tasks queue (or job queue)
1. Tasks queue
The Event Loop parks the asynchronous JavaScript tasks requested by the browser on the task queue or call back queue. This can be a setTimeout, an onClick, or a similar action. The Event Loop loops around and executes a task during each revolution if no other code is being run in the browser. This execution takes place at a specific moment of the loop. This is important, as this determines the order in which the other queues are executed. Make sure to take a look at this bit of the session presented by Jake Archibald in which he illustrates the concept of the Event Loop and the task queue.
2. Render steps
Anything processed on the task queue must also be visualised. This is done using the render steps. This queue comes after the tasks – even though before or after do not really exist in a loop – and consists of four steps:
- Processing requestAnimationFrame
- Style calculations in accordance with the CSS
- Layout rendering: where on the page comes what?
- Painting: creating the pixel data
Just like the order and timing of the queues, the order of these steps is important to remember in order to understand and identify specific issues or bugs.
3. Micro-tasks queue
JavaScript introduced mutation events to monitor certain changes. These later turned into mutation observers and the promise function. A new queue was created for these types of matters: the micro-tasks queue (or job queue). Even though it has a fixed place in the loop, you will not often encounter this queue there. The micro-tasks queue is executed if there is no other JavaScript event being executed in the Event Loop, usually after the completion of a task.
HOW EACH QUEUE IS PROCESSED
It is important to understand how each queue in the Event Loop is processed to be able to identify errors. This is the case because the Loop handles each queue differently.
- The items on the task queue are processed one by one according to the FIFO principle: First In, First Out. The Event Loop continues with the other queues after processing a task. The next task will only be picked up if there is no longer anything to do.
- The render steps are different. All tasks that are in the queue at the moment of processing are completed at once based on the FIFO principle. New tasks that are added during the processing must wait for the next revolution of the Event Loop.
- The micro-tasks queue has yet another approach. The FIFO principle applies again, and all pending tasks are completed before the Event Loop continues, just like the render steps. But you must keep in mind that the Event Loop continues to process micro-tasks until they have all been completed. If you continue to add micro-tasks during the processing, the Event Loop will continue to work on this queue and not do anything else.
WHY IS IT IMPORTANT TO UNDERSTAND THE ORDER AND THE PROCESSING OPERATIONS?
You may wonder why you need this information. The answer is simple: you will write better code with a smaller chance of bugs. If an occasional error does occur, you will find a solution faster because you understand what is happening in the background. We will illustrate this with a few examples.
– Order of the queues
Imagine a button with an onClick element that initiates a regular endless JavaScript loop. Your Event Loop is instructed to execute this task. Usually, the task will be executed and the Loop will continue with the render steps to make the changes to the task visual. But the task addressed by the Loop does not have an end – it is an endless loop. This means that the Event Loop is stuck in the task queue and will never proceed to the render steps. Your web page will freeze and will no longer respond as a result.
– Processing the queues
Because each queue does things differently, issues can also arise at this point if you do not follow the processing order as it should. If you code micro-tasks that keep creating new micro-tasks, your web page will crash. Why? Because the Event Loop continues to process the tasks on the micro-tasks queue until it is empty. This is unlike the task queue, where the Event Loop continues after processing a task. As a result, the Event Loop continues to process an endless series of micro-tasks and never proceeds to the other queues.
Numerous other examples can be mentioned that illustrate the importance of knowing the order and processing operations of the Event Loop. Jake Archibald, who we have cited above, visualises a number of them in his talk at JSConf.Asia. Make sure to take a look at it if you are looking for more examples.
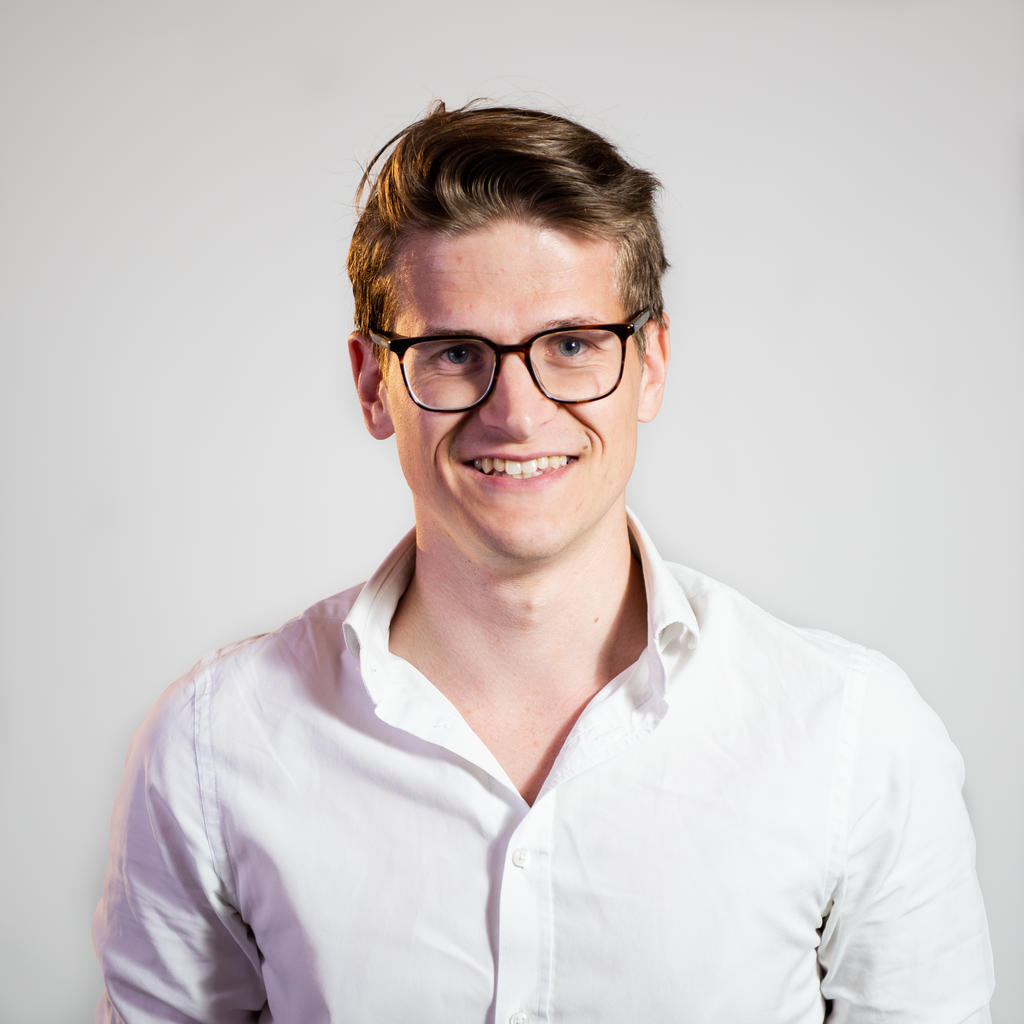